EdgeSmith
Added in version 1.4.
Purpose of this Module
This is a companion module to the ezdxf.edgeminer
module:
create
Edge
instances from DXF primitives for processing by theedgeminer
module:create
LWPolyline
andPolyline
entities from a sequence ofEdge
objects.create
Hatch
boundary paths from a sequence ofEdge
objects.create
ezdxf.path.Path
objects from a sequence ofEdge
objects.
See also
Important
This is the reference documentation and not a tutorial how to use this module.
Make Edges
This functions convert open shapes into 2D edges, closed shapes as circles, closed
arcs, closed ellipses, closed splines and closed polylines are ignored or return
None
.
- ezdxf.edgesmith.make_edge_2d(entity: DXFEntity, *, gap_tol=GAP_TOL) ezdxf.edgeminer.Edge | None
Makes an
Edge
instance from the following DXF entity types:Line
(length accurate)Arc
(length accurate)Ellipse
(length approximated)Spline
(length approximated as straight lines between control points)LWPolyline
(length of bulges as straight line from start- to end point)Polyline
(length of bulges as straight line from start- to end point)
The start- and end points of the edge is projected onto the xy-plane. Returns
None
if the entity has a closed shape or cannot be represented as an edge.
Build From Edges
ARC, LWPOLYLINE, POLYLINE and ELLIPSE entities must have an extrusion vector
of (0, 0, 1) (WCS Z-Axis). Entities with an inverted extrusion vector (0, 0, -1) will be
treated as a 3D curve and approximated by a polyline projected onto the xy-plane.
The ezdxf.upright
module can convert such inverted extrusion vectors to (0, 0, 1).
Curve Approximation
For some target entities curves have to be approximated by polylines.
This process is also called flattening and is controlled by the parameter
max_sagitta
. (Wikipedia)
The max_sagitta
argument defines the maximum distance from the center of the
curve segment to the center of the line segment between two approximation points to
determine if a segment should be subdivided. The default value is -1 and uses a 1/100
of the approximated length of the curve as max_sagitta
.
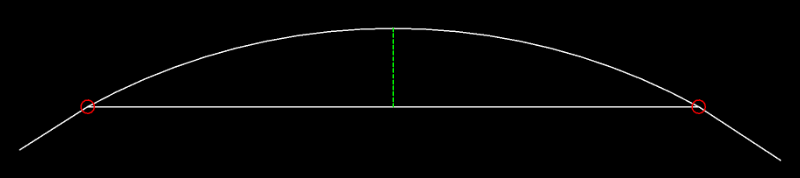
The good choice for a max_sagitta
depends on the output resolution and is maybe
not known at the time of processing the data. E.g. for a printer/plotter a
max_sagitta
of around 1/300 inch (300 dpi) is maybe a good choice.
The convertion from output units to drawing units depends on the scale and is the task
of the package user.
All flattened curves will be projected onto the xy-plane of the WCS.
- ezdxf.edgesmith.lwpolyline_from_chain(edges: Sequence[Edge], *, dxfattribs: Any = None, max_sagitta: float = -1) LWPolyline
Returns a new virtual
LWPolyline
entity.This function assumes the building blocks as simple DXF entities attached as payload to the edges. The edges are processed in order of the input sequence and the start- and end points of the edges should be connected. The output polyline is projected onto the xy-plane of the WCS.
- ezdxf.edgesmith.polyline2d_from_chain(edges: Sequence[Edge], *, dxfattribs: Any = None, max_sagitta: float = -1) Polyline
Returns a new virtual
Polyline
entity.This function assumes the building blocks as simple DXF entities attached as payload to the edges. The edges are processed in order of the input sequence and the start- and end points of the edges should be connected. The output polyline is projected onto the xy-plane of the WCS.
- ezdxf.edgesmith.edge_path_from_chain(edges: Sequence[Edge], *, max_sagitta: float = -1, flags: int = 1) EdgePath
Returns a new
EdgePath
forHatch
entities.This function assumes the building blocks as simple DXF entities attached as payload to the edges. The edges are processed in order of the input sequence and the start- and end points of the edges should be connected. The output path is projected onto the xy-plane of the WCS.
Spline
asSplineEdge
LWPolyline
andPolyline
will be exploded and added asLineEdge
andArcEdge
Everything else will be added as line segment from
Edge.start
toEdge.end
Gaps between edges are connected by line segments.
- Parameters:
edges – Sequence of
Edge
instances with DXF primitives attached as payloadmax_sagitta (float) – curve flattening parameter
flags (int) – default(0), external(1), derived(4), textbox(8) or outermost(16)
- ezdxf.edgesmith.polyline_path_from_chain(edges: Sequence[Edge], *, max_sagitta: float = -1, is_closed=True, flags: int = 1) PolylinePath
Returns a new
PolylinePath
forHatch
entities.This function assumes the building blocks as simple DXF entities attached as payload to the edges. The edges are processed in order of the input sequence and the start- and end points of the edges should be connected. The output path is projected onto the xy-plane of the WCS.
- Parameters:
edges – Sequence of
Edge
instances with DXF primitives attached as payloadmax_sagitta (float) – curve flattening parameter
is_closed (bool) –
True
if path is implicit closedflags (int) – default(0), external(1), derived(4), textbox(8) or outermost(16)
- ezdxf.edgesmith.path2d_from_chain(edges: Sequence[Edge]) Path
Returns a new
ezdxf.path.Path
entity.This function assumes the building blocks as simple DXF entities attached as payload to the edges. The edges are processed in order of the input sequence and the start- and end points of the edges should be connected. The output is a 2D path projected onto the xy-plane of the WCS.
Line
as line segmentArc
as cubic Bézier curvesEllipse
as cubic Bézier curvesSpine
cubic Bézier curvesLWPolyline
andPolyline
as line segments and cubic Bézier curvesEverything else will be added as line segment from
Edge.start
toEdge.end
Gaps between edges are connected by line segments.
Helper Functions
- ezdxf.edgesmith.is_closed_entity(entity: DXFEntity) bool
Returns
True
if the given entity represents a closed loop.Tests the following DXF entities:
CIRCLE
ARC
ELLIPSE
SPLINE
LWPOLYLINE
POLYLINE
HATCH
SOLID
TRACE
Returns
False
for all other DXF entities.
- ezdxf.edgesmith.is_pure_2d_entity(entity: DXFEntity) bool
Returns
True
if the given entity represents a pure 2D entity in the xy-plane of the WCS.All vertices must be in the xy-plane of the WCS.
Thickness must be 0.
The extrusion vector must be (0, 0, 1).
Entities with inverted extrusions vectors (0, 0, -1) are not pure 2D entities. The
ezdxf.upright
module can be used to revert inverted extrusion vectors back to (0, 0, 1).
Tests the following DXF entities:
LINE
CIRCLE
ARC
ELLIPSE
SPLINE
LWPOLYLINE
POLYLINE
HATCH
SOLID
TRACE
Returns
False
for all other DXF entities.
- ezdxf.edgesmith.filter_edge_entities(entities: Iterable[DXFEntity]) Iterator[DXFEntity]
Returns all entities that can be used to build edges in the context of
ezdxf.edgeminer
.Returns the following DXF entities:
LINE
ARC
ELLIPSE
SPLINE
LWPOLYLINE
POLYLINE
Note
CIRCLE, TRACE and SOLID are closed shapes by definition and cannot be used as edge in the context of
ezdxf.edgeminer
orezdxf.edgesmith
.This filter is not limited to pure 2D entities!
Does not test if the entity is a closed loop!
- ezdxf.edgesmith.filter_2d_entities(entities: Iterable[DXFEntity]) Iterator[DXFEntity]
Returns all pure 2D entities, ignores all entities placed outside or extending beyond the xy-plane of the WCS. See
is_pure_2d_entity()
for more information.
- ezdxf.edgesmith.filter_open_edges(entities: Iterable[DXFEntity]) Iterator[DXFEntity]
Returns all open linear entities usable as edges in the context of
ezdxf.edgeminer
orezdxf.edgesmith
.Ignores all entities that represent closed loops like circles, closed arcs, closed ellipses, closed splines and closed polylines.
Note
This filter is not limited to pure 2D entities!
- ezdxf.edgesmith.bounding_box_2d(edges: Sequence[Edge]) BoundingBox2d
Returns the
BoundingBox2d
of all start- and end vertices.
- ezdxf.edgesmith.loop_area(edges: Sequence[Edge], *, gap_tol=GAP_TOL) float
Returns the area of a closed loop.
- Raises:
ValueError – edges are not a closed loop
- ezdxf.edgesmith.is_inside_polygon_2d(edges: Sequence[Edge], point: UVec, *, gap_tol=GAP_TOL) bool
Returns
True
when point is inside the polygon.The edges must be a closed loop. The polygon is build from edges as straight lines from start- to end vertex, independently whatever the edges really represent. A point at a boundary line is inside the polygon.
- Parameters:
edges – sequence of
Edge
representing a closed looppoint – point to test
gap_tol – maximum vertex distance to consider two edges as connected
- Raises:
ValueError – edges are not a closed loop
- ezdxf.edgesmith.intersecting_edges_2d(edges: Sequence[em.Edge], p1: UVec, p2: UVec | None = None) list[IntersectingEdge]
Returns all edges that intersect a line from point p1 to point p2.
If p2 is
None
an arbitrary point outside the bounding box of all start- and end vertices beyond extmax.x will be chosen. The edges are handled as straight lines from start- to end vertex, projected onto the xy-plane of the WCS. The result is sorted by the distance from intersection point to point p1.
- class ezdxf.edgesmith.IntersectingEdge
NamedTuple as return type of
intersecting_edges_2d()
.- distance
Distance from intersection point to point p1 as float; alias for field 1.
Global Constants
GAP_TOL = 1e-9
LEN_TOL = 1e-9 # length and distance
DEG_TOL = 1e-9 # angles in degree
RAD_TOL = 1e-7 # angles in radians