Tutorial for PolyfaceΒΆ
The Polyface
entity represents a 3D mesh build of
vertices and faces and is just an extended POLYLINE entity with a complex
VERTEX structure. The Polyface
entity was used in DXF R12 and older
DXF versions and is still supported by newer DXF versions. The new
Mesh
entity stores the same data much more efficient
but requires DXF R2000 or newer. The Polyface
entity supports only
triangles and quadrilaterals as faces, the Mesh
entity supports also
n-gons.
Its recommended to use the MeshBuilder
objects to
create 3D meshes and render them as POLYFACE entities by the
render_polymesh()
method into a layout:
import ezdxf
from ezdxf import colors
from ezdxf.gfxattribs import GfxAttribs
from ezdxf.render import forms
cube = forms.cube().scale_uniform(10).subdivide(2)
red = GfxAttribs(color=colors.RED)
green = GfxAttribs(color=colors.GREEN)
blue = GfxAttribs(color=colors.BLUE)
doc = ezdxf.new()
msp = doc.modelspace()
# render as MESH entity
cube.render_mesh(msp, dxfattribs=red)
cube.translate(20)
# render as POLYFACE a.k.a. POLYLINE entity
cube.render_polyface(msp, dxfattribs=green)
cube.translate(20)
# render as a bunch of 3DFACE entities
cube.render_3dfaces(msp, dxfattribs=blue)
doc.saveas("meshes.dxf")
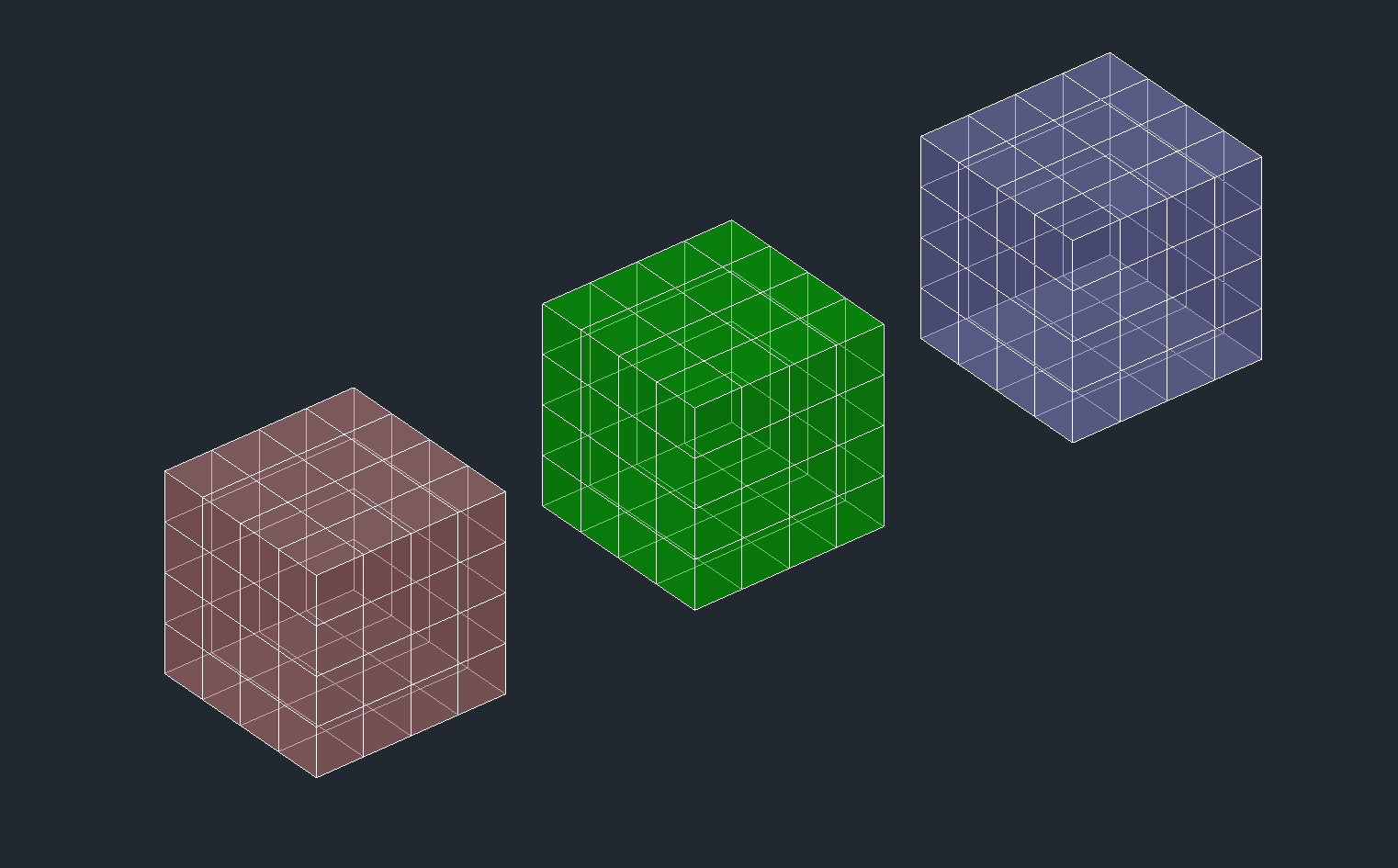
Warning
If the mesh contains n-gons the render methods for POLYFACE and 3DFACES subdivides the n-gons into triangles, which does not work for concave faces.
The usage of the MeshBuilder
object is also recommended
for inspecting Polyface
entities:
MeshBuilder.vertices
is a sequence of 3D points asezdxf.math.Vec3
objectsa face in
MeshBuilder.faces
is a sequence of indices into theMeshBuilder.vertices
sequence
import ezdxf
from ezdxf.render import MeshBuilder
def process(mesh):
# vertices is a sequence of 3D points
vertices = mses.vertices
# a face is a sequence of indices into the vertices sequence
faces = mesh.faces
...
doc = ezdxf.readfile("meshes.dxf")
msp = doc.modelspace()
for polyline in msp.query("POLYLINE"):
if polyline.is_poly_face_mesh:
mesh = MeshBuilder.from_polyface(polyline)
process(mesh)
See also