Tutorial for Entity Selection¶
This tutorial shows how to use the ezdxf.select
module, which provides functions
to select entities based on various shapes. These selection functions offer a way to
filter entities based on their spatial location.
This is the base document for this tutorial:
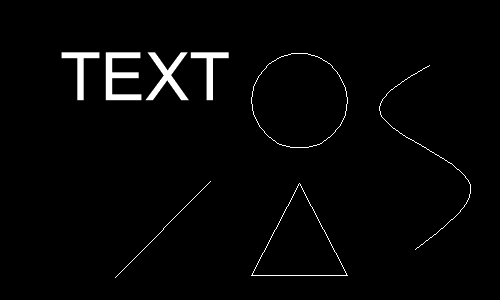
Why Bounding Boxes?¶
The select
module primarily relies on bounding boxes to perform selections.
Bounding boxes offer a fast way to identify potential overlaps between entities and the
selection shape. This approach prioritizes performance over absolute accuracy.
Note
The bounding boxes for text-based entities and entities containing curves are not
accurate! For more information read the docs for the ezdxf.bbox
module.
Source of Entities¶
The source of the selection can be any iterable of DXF entities, like the modelspace,
any paperspace layout or a block layout, also the result of an entity query as an
EntityQuery
container, or any collection of DXF entities that
implements the __iter__()
method.
Selection Shapes¶
Using Selection Functions¶
These selection functions utilize the selection shapes:
bbox_inside()
: Selects entities whose bounding box lies withing the selection shape.bbox_outside()
: Selects entities whose bounding box is completely outside the selection shape.bbox_overlap()
: Selects entities whose bounding box overlaps the selection shape.
Additional selection functions:
bbox_chained()
: Selects entities that are linked together by overlapping bounding boxes.bbox_crosses_fence()
: Selects entities whose bounding box overlaps an open polyline.point_in_bbox()
: Selects entities where the selection point lies within the bounding box.
The functions return an EntityQuery
object, which provides access
to the selected entities. You can iterate over the EntityQuery
to access each
selected entity.
Bounding Box Inside Selection¶
Selects entities which bounding boxes are completely within the selection shape.
Example to select entities inside a window:
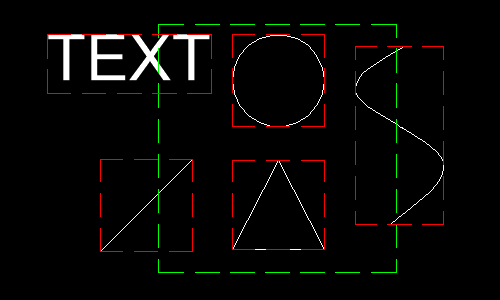
import ezdxf
from ezdxf import select
doc = ezdxf.readfile("base.dxf")
msp 0 doc.modelspace()
window = select.Window((150, 105), (280, 240))
for entity in select.bbox_inside(window, msp):
print(str(entity))
output:
CIRCLE(#9D)
LWPOLYLINE(#9E)
Bounding Box Outside Selection¶
Selects entities whose bounding box is completely outside the selection shape.
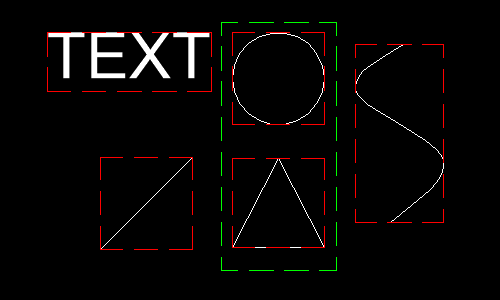
window = select.Window((185, 105), (245, 240))
for entity in select.bbox_outside(window, msp):
print(str(entity))
output:
TEXT(#9F)
SPLINE(#A0)
LINE(#A1)
Bounding Box Overlap Selection¶
Selects entities whose bounding box overlaps the selection shape.
This function works similar to the crossing selection in CAD applications, but not exactly the same. The function selects entities whose bounding boxes overlap the selection shape. This will also select elements where all of the entity geometry is outside the selection shape, but the bounding box overlaps the selection shape, e.g. border polylines.
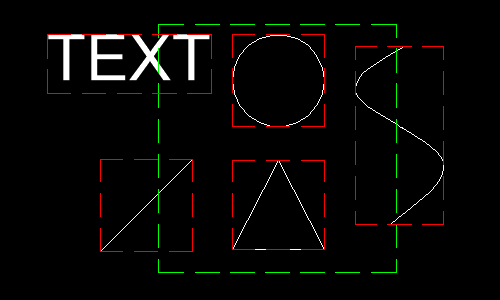
window = select.Window((150, 105), (280, 240))
for entity in select.bbox_overlap(window, msp):
print(str(entity))
output:
CIRCLE(#9D)
LWPOLYLINE(#9E)
TEXT(#9F)
SPLINE(#A0)
LINE(#A1)
LWPOLYLINE(#A2)
Bounding Box Chained Selection¶
Selects elements that are directly or indirectly connected to each other by overlapping bounding boxes. The selection begins at the specified starting element.
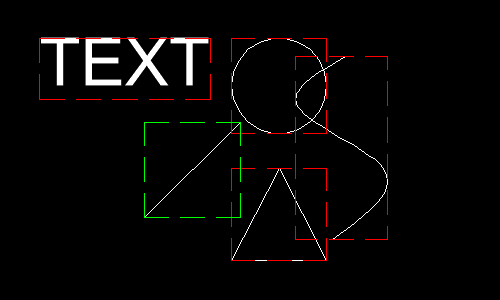
# choose entity for the beginning of the chain:
line = msp.query("LINE").first
for entity in select.bbox_chained(line, msp):
print(str(entity))
output:
LINE(#A1)
CIRCLE(#9D)
LWPOLYLINE(#9E)
SPLINE(#A0)
Bounding Box Crosses Fence¶
Selects entities whose bounding box intersects an open polyline.
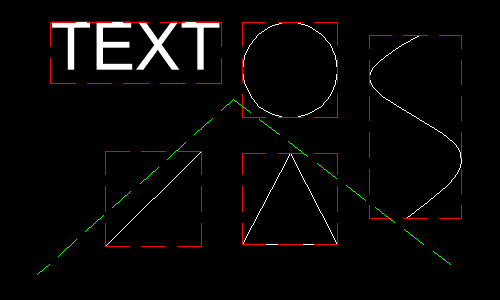
for entity in select.bbox_crosses_fence([(83, 101), (186, 193), (300, 107)], msp):
print(str(entity))
output:
CIRCLE(#9D)
LWPOLYLINE(#9E)
SPLINE(#A0)
LINE(#A1)
Note
The polyline does not cross the entity geometry itself!
Point In Bounding Box Selection¶
Selects entities where the selection point lies within the bounding box.
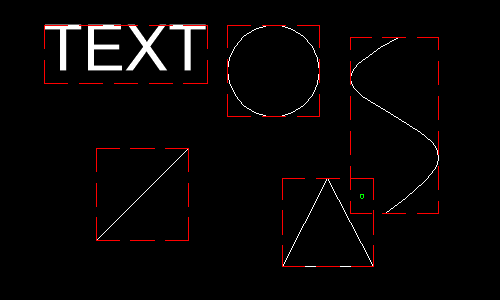
for entity in select.bbox_point((264, 140), msp):
print(str(entity))
output:
LWPOLYLINE(#9E)
SPLINE(#A0)
Circle Selection¶
For the circle shape, the selection tests are carried out on the real circlar area.
This example selects all entities around the CIRCLE entity within a 60 unit radius whose bounding box overlaps the circle selection:
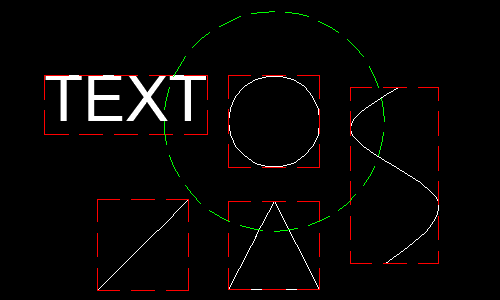
entity = msp.query("CIRCLE").first
circle = select.Circle(entity.dxf.center, radius=60)
for entity in select.bbox_overlap(circle, msp):
print(str(entity))
output:
CIRCLE(#9D)
LWPOLYLINE(#9E)
TEXT(#9F)
SPLINE(#A0)
Polygon Selection¶
As for the circle shape, the polygon selection tests are carried out on the real polygon area.
Note
This may not work 100% correctly if the selection polygon has a complex concave shape!
This example selects all entities whose bounding box lies entirely within the selection polygon:
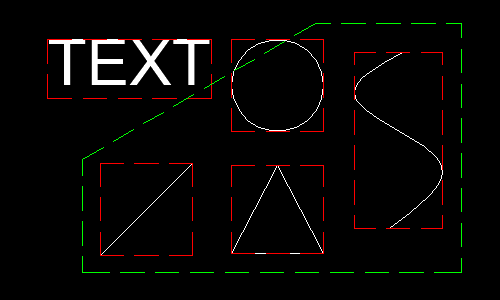
polygon = select.Polygon([(110, 168), (110, 107), (316, 107), (316, 243), (236, 243)])
for entity in select.bbox_inside(polygon, msp):
print(str(entity))
output:
LWPOLYLINE(#9E)
SPLINE(#A0)
LINE(#A1)