OpenSCAD
Interface to the OpenSCAD application to apply boolean operations to
MeshBuilder
objects. For more information about boolean
operations read the documentation of OpenSCAD. The OpenSCAD application is
not bundled with ezdxf, you need to install the application yourself.
On Windows the path to the openscad.exe
executable is
stored in the config file (see ezdxf.options
) in the “openscad-addon”
section as key “win_exec_path”, the default entry is:
[openscad-addon]
win_exec_path = "C:\Program Files\OpenSCAD\openscad.exe"
On Linux and macOS the openscad
command is located by the
shutil.which()
function.
Example:
import ezdxf
from ezdxf.render import forms
from ezdxf.addons import MengerSponge, openscad
doc = ezdxf.new()
msp = doc.modelspace()
# 1. create the meshes:
sponge = MengerSponge(level=3).mesh()
sponge.flip_normals() # important for OpenSCAD
sphere = forms.sphere(
count=32, stacks=16, radius=0.5, quads=True
).translate(0.25, 0.25, 1)
sphere.flip_normals() # important for OpenSCAD
# 2. create the script:
script = openscad.boolean_operation(openscad.DIFFERENCE, sponge, sphere)
# 3. execute the script by OpenSCAD:
result = openscad.run(script)
# 4. render the MESH entity:
result.render_mesh(msp)
doc.set_modelspace_vport(6, center=(5, 0))
doc.saveas("OpenSCAD.dxf")
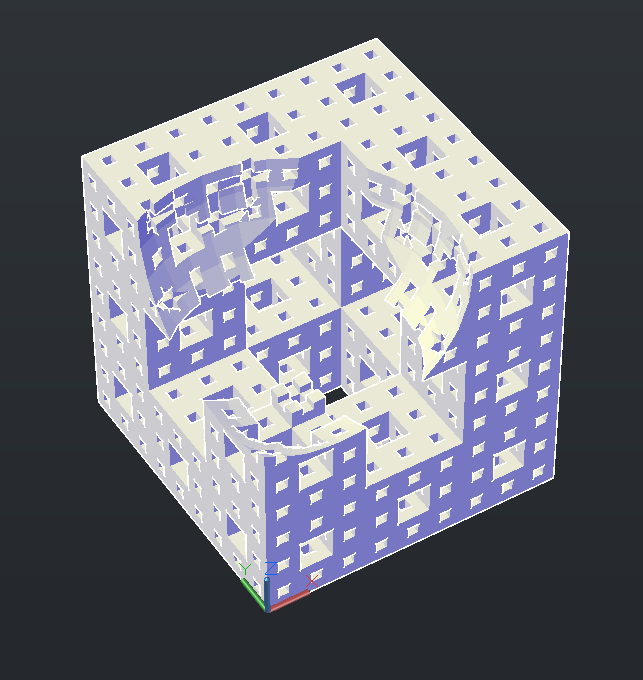
Functions
- ezdxf.addons.openscad.run(script: str, exec_path: str | None = None) MeshTransformer
Executes the given script by OpenSCAD and returns the result mesh as
MeshTransformer
.- Parameters:
script – the OpenSCAD script as string
exec_path – path to the executable as string or
None
to use the default installation path
- ezdxf.addons.openscad.boolean_operation(op: Operation, mesh1: MeshBuilder, mesh2: MeshBuilder) str
Returns an OpenSCAD script to apply the given boolean operation to the given meshes.
The supported operations are:
UNION
DIFFERENCE
INTERSECTION
- ezdxf.addons.openscad.is_installed() bool
Returns
True
if OpenSCAD is installed.Searches on Windows the path stored in the options as “win_exec_path” in section “[openscad-addon]” which is “C:Program FilesOpenSCADopenscad.exe” by default.
Searches the “openscad” command on Linux and macOS.
Script Class
- class ezdxf.addons.openscad.Script
Helper class to build OpenSCAD scripts. This is a very simple string building class and does no checks at all! If you need more advanced features to build OpenSCAD scripts look at the packages solidpython2 and openpyscad.
- add(data: str) None
Add a string.
- add_mirror(v: UVec) None
Add a
mirror()
operation.OpenSCAD docs: https://en.wikibooks.org/wiki/OpenSCAD_User_Manual/Transformations#mirror
- Parameters:
v – the normal vector of a plane intersecting the origin through which to mirror the object
- add_multmatrix(m: Matrix44) None
Add a transformation matrix of type
Matrix44
asmultmatrix()
operation.OpenSCAD docs: https://en.wikibooks.org/wiki/OpenSCAD_User_Manual/Transformations#multmatrix
- add_polyhedron(mesh: MeshBuilder) None
Add mesh as
polyhedron()
command.OpenSCAD docs: https://en.wikibooks.org/wiki/OpenSCAD_User_Manual/Primitive_Solids#polyhedron
- add_polygon(path: Iterable[UVec], holes: Sequence[Iterable[UVec]] | None = None) None
Add a
polygon()
command. This is a 2D command, all z-axis values of the input vertices are ignored and all paths and holes are closed automatically.OpenSCAD docs: https://en.wikibooks.org/wiki/OpenSCAD_User_Manual/Using_the_2D_Subsystem#polygon
- Parameters:
path – exterior path
holes – a sequence of one or more holes as vertices, or
None
for no holes
- add_resize(nx: float, ny: float, nz: float, auto: bool | tuple[bool, bool, bool] | None = None) None
Add a
resize()
operation.OpenSCAD docs: https://en.wikibooks.org/wiki/OpenSCAD_User_Manual/Transformations#resize
- Parameters:
nx – new size in x-axis
ny – new size in y-axis
nz – new size in z-axis
auto – If the auto argument is set to
True
, the operation auto-scales any 0-dimensions to match. Set the auto argument as a 3-tuple of bool values to auto-scale individual axis.
- add_rotate(ax: float, ay: float, az: float) None
Add a
rotation()
operation.OpenSCAD docs: https://en.wikibooks.org/wiki/OpenSCAD_User_Manual/Transformations#rotate
- Parameters:
ax – rotation about the x-axis in degrees
ay – rotation about the y-axis in degrees
az – rotation about the z-axis in degrees
- add_rotate_about_axis(a: float, v: UVec) None
Add a
rotation()
operation about the given axis v.OpenSCAD docs: https://en.wikibooks.org/wiki/OpenSCAD_User_Manual/Transformations#rotate
- Parameters:
a – rotation angle about axis v in degrees
v – rotation axis as
ezdxf.math.UVec
object
- add_scale(sx: float, sy: float, sz: float) None
Add a
scale()
operation.OpenSCAD docs: https://en.wikibooks.org/wiki/OpenSCAD_User_Manual/Transformations#scale
- Parameters:
sx – scaling factor for the x-axis
sy – scaling factor for the y-axis
sz – scaling factor for the z-axis
- add_translate(v: UVec) None
Add a
translate()
operation.OpenSCAD docs: https://en.wikibooks.org/wiki/OpenSCAD_User_Manual/Transformations#translate
- Parameters:
v – translation vector
- get_string() str
Returns the OpenSCAD build script.
Boolean Operation Constants
- ezdxf.addons.openscad.UNION
- ezdxf.addons.openscad.DIFFERENCE
- ezdxf.addons.openscad.INTERSECTION
openpyscad
This add-on is not a complete wrapper around OpenSCAD, if you need such a tool look at the openpyscad or solidpython2 packages at PyPI.
Not sure if the openpyscad package is still maintained, the last commit at github is more than a year old and did not pass the CI process! (state June 2022)
This code snippet shows how to get the MeshTransformer
object from the basic openpyscad example:
from ezdxf.addons import openscad
import openpyscad as ops
c1 = ops.Cube([10, 20, 10])
c2 = ops.Cube([20, 10, 10])
# dump OpenSCAD script as string:
script = (c1 + c2).dumps()
# execute script and load the result as MeshTransformer instance:
mesh = openscad.run(script)
Create an openpyscad Polyhedron
object from an ezdxf
MeshBuilder
object:
from ezdxf.render import forms
import openpyscad as ops
# create an ezdxf MeshBuilder() object
sphere = forms.sphere()
sphere.flip_normals() # required for OpenSCAD
# create an openpyscad Polyhedron() object
polyhedron = ops.Polyhedron(
points=[list(p) for p in sphere.vertices], # convert Vec3 objects to lists!
faces=[list(f) for f in sphere.faces], # convert face tuples to face lists!
)
# create the OpenSCAD script:
script = polyhedron.dumps()
The type conversion is needed to get valid OpenSCAD code from openpyscad!
solidpython2
The solidpython2 package seems to be better maintained than the openpyscad package, but this is just an opinion based on newer commits at github (link) for the solidpython2 package.
Same example for solidpython2:
from ezdxf.addons import openscad
from solid2 import cube, scad_render
c1 = cube([10, 20, 10])
c2 = cube([20, 10, 10])
# dump OpenSCAD script as string:
script = scad_render(c1 + c2)
# execute script and load the result as MeshTransformer instance:
mesh = openscad.run(script)
Create a solidpython2 polyhedron
object from an ezdxf
MeshBuilder
object:
from ezdxf.render import forms
from solid2 import polyhedron, scad_render
# create an ezdxf MeshBuilder() object
sphere = forms.sphere()
sphere.flip_normals() # required for OpenSCAD
# create a solidpython2 polyhedron() object
ph = polyhedron(
points=[v.xyz for v in sphere.vertices], # convert Vec3 objects to tuples!
faces=sphere.faces, # types are compatible
)
# create the OpenSCAD script:
script = scad_render(ph)