General Document¶
General preconditions:
import sys
import ezdxf
try:
doc = ezdxf.readfile("your_dxf_file.dxf")
except IOError:
print(f"Not a DXF file or a generic I/O error.")
sys.exit(1)
except ezdxf.DXFStructureError:
print(f"Invalid or corrupted DXF file.")
sys.exit(2)
msp = doc.modelspace()
This works well with DXF files from trusted sources like AutoCAD or BricsCAD,
for loading DXF files with minor or major flaws look at the
ezdxf.recover
module.
Load DXF Files with Structure Errors¶
If you know the files you will process have most likely minor or major flaws,
use the ezdxf.recover
module:
import sys
from ezdxf import recover
try: # low level structure repair:
doc, auditor = recover.readfile(name)
except IOError:
print(f"Not a DXF file or a generic I/O error.")
sys.exit(1)
except ezdxf.DXFStructureError:
print(f"Invalid or corrupted DXF file: {name}.")
sys.exit(2)
# DXF file can still have unrecoverable errors, but this is maybe
# just a problem when saving the recovered DXF file.
if auditor.has_errors:
print(f"Found unrecoverable errors in DXF file: {name}.")
auditor.print_error_report()
For more loading scenarios follow the link: ezdxf.recover
Set/Get Header Variables¶
ezdxf has an interface to get and set HEADER variables:
doc.header["VarName"] = value
value = doc.header["VarName"]
See also
HeaderSection
and online documentation from Autodesk
for available header variables.
Set DXF Drawing Units¶
The header variable $INSUNITS defines the drawing units for the modelspace and therefore for the DXF document if no further settings are applied. The most common units are 6 for meters and 1 for inches.
Use this HEADER variables to setup the default units for CAD applications opening the DXF file. This setting is not relevant for ezdxf API calls, which are unitless for length values and coordinates and decimal degrees for angles (in most cases).
Sets drawing units:
doc.header["$INSUNITS"] = 6
For more information see section DXF Units.
Explore the DXF File Structure¶
DXF files are plain text files, you can open this files with every text editor which handles bigger files. But it is not really easy to get quick the information you want.
Use the DXF structure browser:
# Call as executable script from the command line:
ezdxf browse FILE
# Call as module on Windows:
py -m ezdxf browse FILE
# Call as module on Linux/Mac
python3 -m ezdxf browse FILE
This command requires PySide6 or PyQt5 to be installed. It opens a desktop window with a selection panel for all DXF entities in the document, and handles int the entity view are links between DXF entities, this simplifies the navigation between the DXF entities. Read the docs for the Browse command for more information.
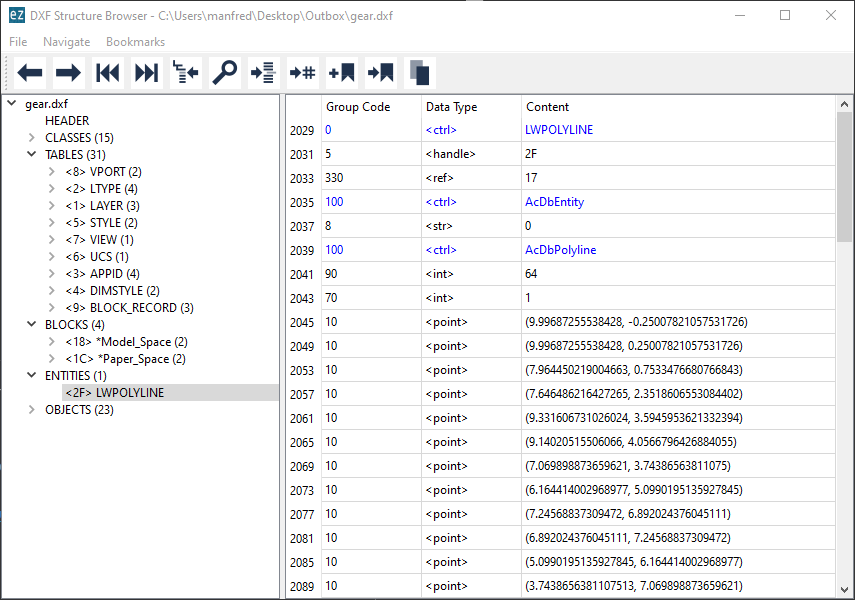
Calculate Extents for the Modelspace¶
Since ezdxf v0.16 exist a ezdxf.bbox
module to calculate bounding
boxes for DXF entities. This module makes the extents calculation very easy,
but read the documentation for the bbox
module to understand its
limitations.
import ezdxf
from ezdxf import bbox
doc = ezdxf.readfile("your.dxf")
msp = doc.modelspace()
extents = bbox.extents(msp)
The returned extents is a ezdxf.math.BoundingBox
object.
Set Initial View/Zoom for the Modelspace¶
To show an arbitrary location of the modelspace centered in the CAD application
window, set the '*Active'
VPORT to this location. The DXF attribute
dxf.center
defines the location in the modelspace, and the dxf.height
specifies the area of the modelspace to view. Shortcut function:
doc.set_modelspace_vport(height=10, center=(10, 10))
See also
The ezdxf.zoom
module is another way to set the initial modelspace
view.
Setting the initial view to the extents of all entities in the modelspace:
import ezdxf
from ezdxf import zoom
doc = ezdxf.readfile("your.dxf")
msp = doc.modelspace()
zoom.extents(msp)
Setting the initial view to the extents of just some entities:
lines = msp.query("LINES")
zoom.objects(lines)
The zoom
module also works for paperspace layouts.
Hide the UCS Icon¶
The visibility of the UCS icon is controlled by the DXF
ucs_icon
attribute of the
VPort
entity:
bit 0: 0=hide, 1=show
bit 1: 0=display in lower left corner, 1=display at origin
The state of the UCS icon can be set in conjunction with the initial
VPort
of the model space, this code turns off the UCS
icon:
doc.set_modelspace_vport(10, center=(10, 10), dxfattribs={"ucs_icon": 0})
Alternative: turn off UCS icons for all VPort
entries in the active
viewport configuration:
for vport in doc.viewports.get_config("*Active"):
vport.dxf.ucs_icon = 0
Show Lineweights in DXF Viewers¶
By default lines and curves are shown without lineweights in DXF viewers. By setting the header variable $LWDISPLAY to 1 the DXF viewer should display lineweights, if supported by the viewer.
doc.header["$LWDISPLAY"] = 1
Add ezdxf Resources to Existing DXF Document¶
Add all ezdxf specific resources (line types, text- and dimension styles) to an existing DXF document:
import ezdxf
from ezdxf.tools.standards import setup_drawing
doc = ezdxf.readfile("your.dxf")
setup_drawing(doc, topics="all")
Set Logging Level of ezdxf¶
Set the logging level of the ezdxf package to a higher level to minimize
logging messages from ezdxf. At level ERROR
only severe errors will be
logged and WARNING
, INFO
and DEBUG
messages will be suppressed:
import logging
logging.getLogger("ezdxf").setLevel(logging.ERROR)